Dark Mode in SwiftUI
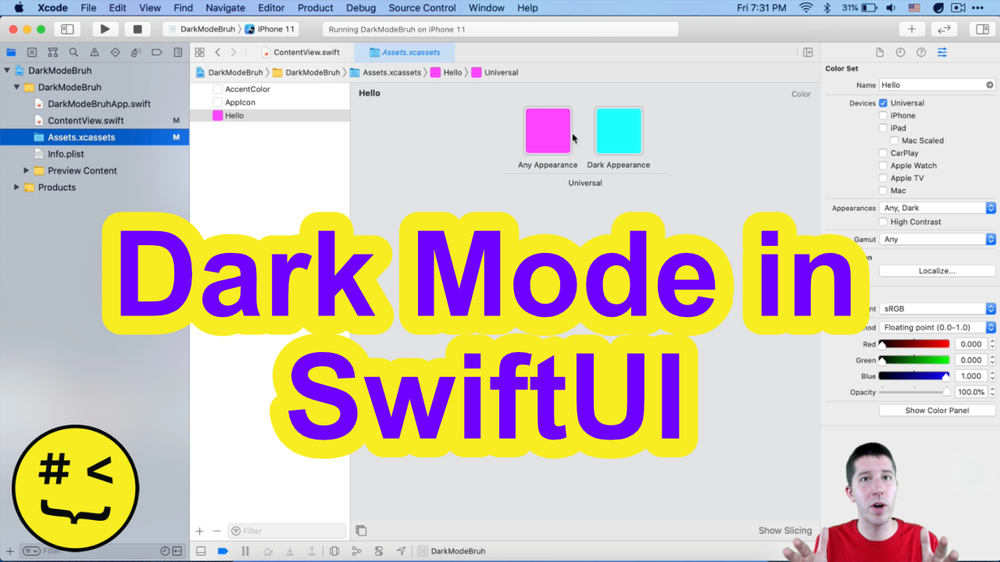
There are several ways to make sure your app looks good in dark mode
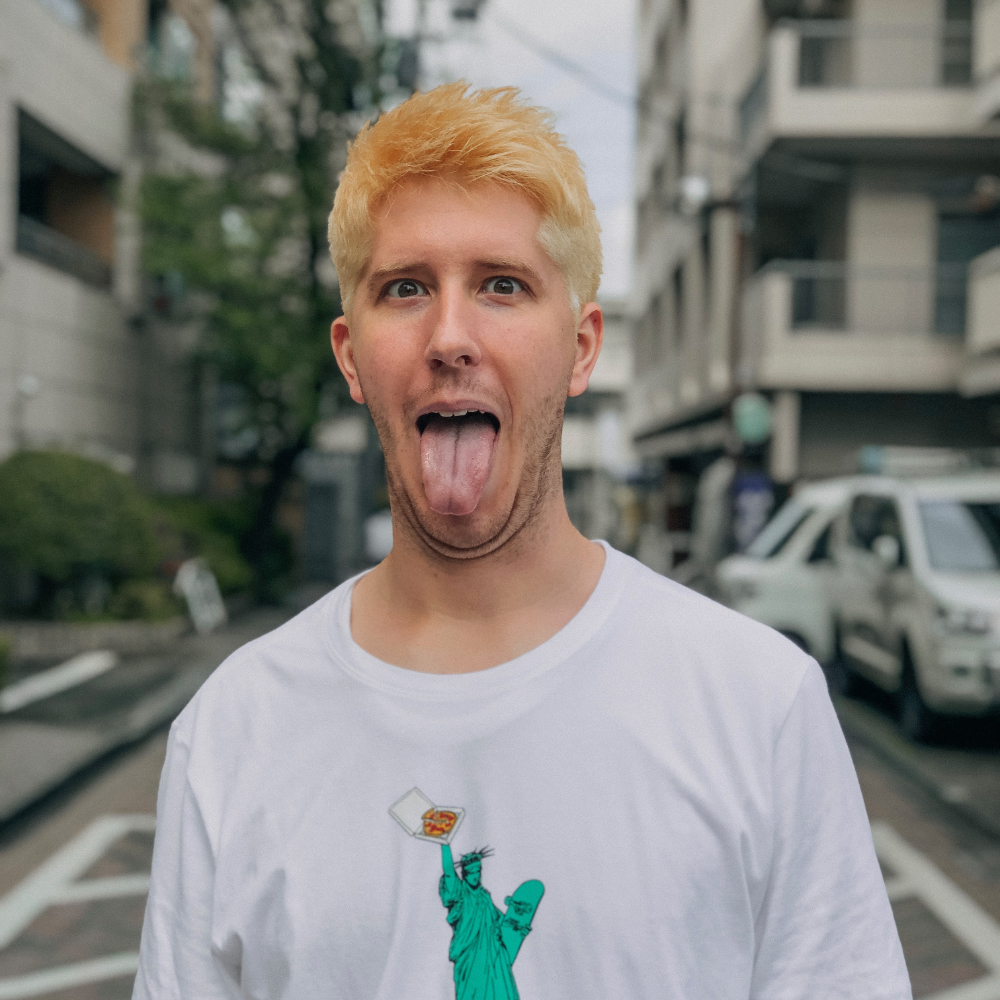
nicksensei
2 min read
Dec 3, 2020
There are several ways to make sure your app looks good in dark mode.
1. Don't Set Foreground Colors
Text views in SwiftUI by default will automatically be black in light mode and white in dark mode. The backgrounds of views are the exact opposite. Don't change colors if you don't need to.
2. Use Color Sets
Got to your Assets.xcassets and create a new Color Set where you can set a color Any Appearance (light mode) and Dark Appearance (Dark Mode). Then in code, when you use that color, it will automatically change the color based on if you are in dark or light mode.
Text("Hello, world!").foregroundColor(colorScheme == .dark ? .red : .blue)
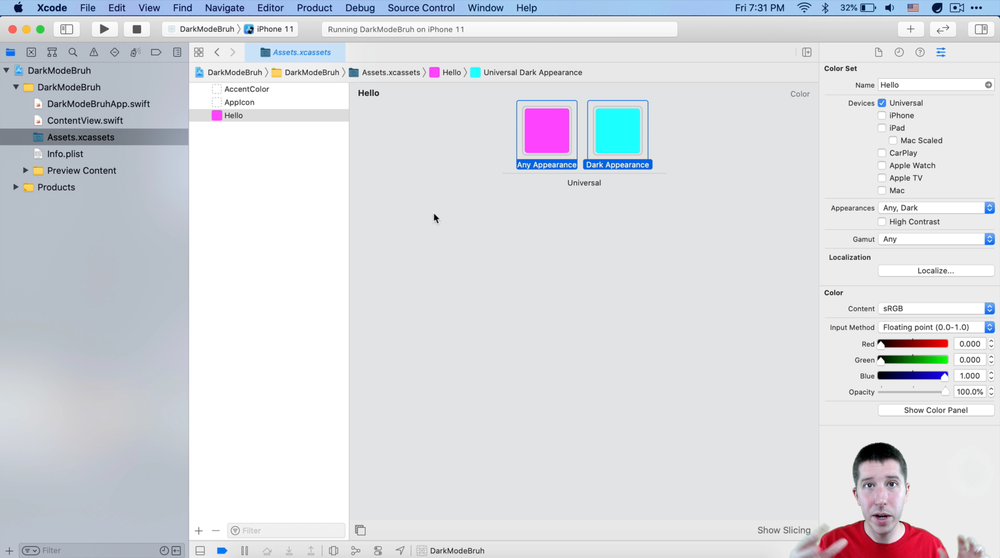
3. Use The colorScheme Environment Variable
Inside your view struct, add the colorScheme environment variable
struct ContentView: View {
@Environment(\.colorScheme) var colorScheme
var body: some View {
...
}
}
Then use that colorScheme variable to make different design decisions like in an if statement:
if colorScheme == .dark {
Rectangle()
} else {
Circle()
}
Or inline for something like a color
Text("Hello, world!").foregroundColor(colorScheme == .dark ? .red : .blue)
If you want to test light and dark mode in your preview so that you don't have to constantly run your app (and so that you can quickly see if changes you are making will look bad in dark mode) first make a Group in your preview. Then on each, set the colorScheme to .light or .dark
Group {
ContentView()
.environment(\.colorScheme, .light)
ContentView()
.environment(\.colorScheme, .dark)
}
Want to learn more SwiftUI?
Check out my new iOS 17 SwiftUI course. Pick your own price and make some awesome apps!
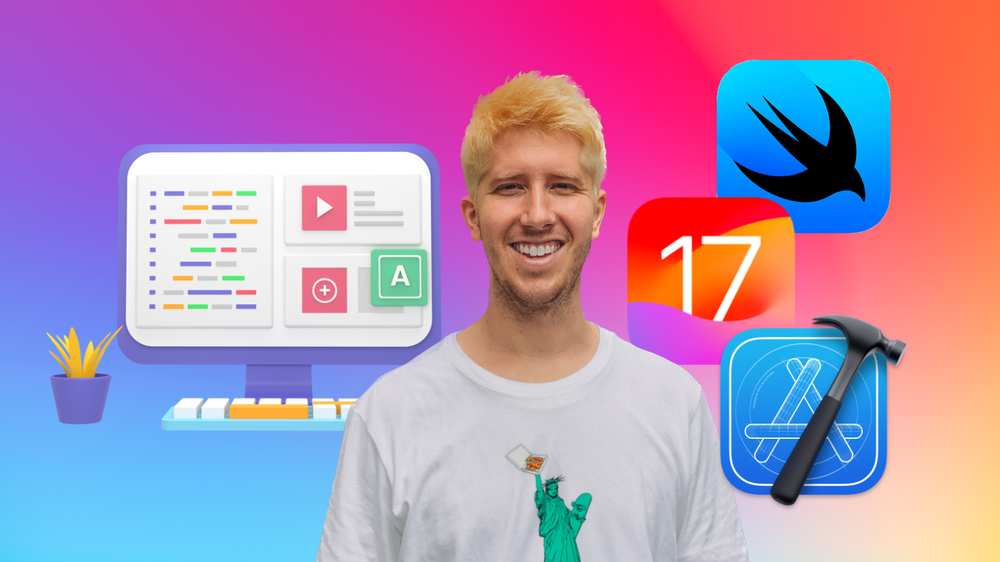